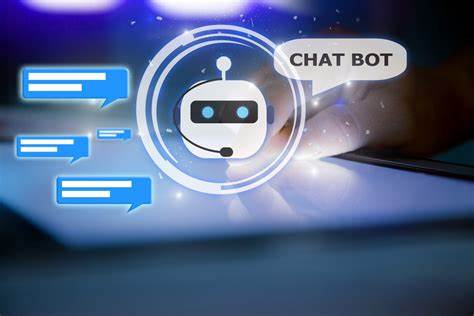
🌟 Want to create your own chatbot? 🌟
- Build a Simple Chatbot in Python in Just 10 Steps!
- Chatbots are everywhere these days, from customer service to personal assistants. But have you ever wondered how they work? Today, we’ll walk you through building a simple chatbot in Python using the Natural Language Toolkit (NLTK). By the end of this tutorial, you’ll have a chatbot that can respond to basic inputs.
- What You’ll Need
- Python 3.x installed on your computer
- A text editor or an IDE (like VS Code or PyCharm)
- Basic understanding of Python programming
- Let’s dive in!
Step 1: Install the Required Library
First, you need to install the NLTK library. NLTK (Natural Language Toolkit) is a powerful library for working with human language data.
Run the following command in your terminal or command prompt:
pip install nltk
Step 2: Import the Necessary Libraries
Start by importing the required modules from the NLTK library.
import nltk
from nltk.chat.util import Chat, reflections
The Chat
module helps us create a chatbot, while reflections
is a dictionary that maps pronouns (like “I” to “you”) to make responses more natural.
Step 3: Define the Chatbot’s Responses
The chatbot’s behavior is defined using a list of pairs. Each pair consists of a regular expression (to match user input) and a list of possible responses.
Here’s an example:
pairs = [
['(hi|hello|hey)', ['Hello! How can I help you today?']],
['(bye|exit)', ['Goodbye! Have a great day!']],
['(.*your name.*)', ['I am your friendly Python chatbot!']],
['(how are you.*)', ['I’m just a bunch of code, but I’m doing great! How about you?']],
['(.*help.*)', ['Sure, I’d be happy to help. What do you need assistance with?']]
]
pairs = [
['(hi|hello|hey)', ['Hello! How can I help you today?']],
['(bye|exit)', ['Goodbye! Have a great day!']],
['(.*your name.*)', ['I am your friendly Python chatbot!']],
['(how are you.*)', ['I’m just a bunch of code, but I’m doing great! How about you?']],
['(.*help.*)', ['Sure, I’d be happy to help. What do you need assistance with?']]
]
This setup allows the chatbot to recognize specific patterns in user input and respond accordingly.
Step 4: Initialize the Chatbot
Create an instance of the Chat
class using the pairs
and reflections
defined earlier.
chatbot = Chat(pairs, reflections)
Step 5: Start the Chat
Now, you’re ready to launch your chatbot! Use the converse()
method to start a conversation.
print("Hi! I am a chatbot. Type 'bye' to exit.")
chatbot.converse()
Complete Code
Here’s the full code for your chatbot:
import nltk
from nltk.chat.util import Chat, reflections
# Define the chatbot's responses
pairs = [
['(hi|hello|hey)', ['Hello! How can I help you today?']],
['(bye|exit)', ['Goodbye! Have a great day!']],
['(.*your name.*)', ['I am your friendly Python chatbot!']],
['(how are you.*)', ['I’m just a bunch of code, but I’m doing great! How about you?']],
['(.*help.*)', ['Sure, I’d be happy to help. What do you need assistance with?']]
]
# Initialize the chatbot
chatbot = Chat(pairs, reflections)
# Start the conversation
print("Hi! I am a chatbot. Type 'bye' to exit.")
chatbot.converse()
How It Works
- Pattern Matching: The chatbot uses regular expressions to match user input to patterns defined in
pairs
. - Reflections: The
reflections
dictionary ensures responses sound more natural by swapping pronouns (e.g., “I” to “you”). - Random Responses: If multiple responses are defined for a pattern, the chatbot randomly selects one, making it feel less repetitive.
Enhancements You Can Make
This chatbot is a great starting point, but there’s so much more you can do:
- Add More Patterns: Expand the
pairs
list to handle more types of user input. - Integrate APIs: Connect the chatbot to APIs for weather updates, jokes, or more.
- Use Machine Learning: Implement a more advanced chatbot using machine learning libraries like TensorFlow or PyTorch.
- Deploy It Online: Host your chatbot on a web application using frameworks like Flask or Django.
What’s Next?
Now that you have a working chatbot, it’s time to get creative! Try customizing the responses or adding new features to make it more interactive and useful.
💬 What kind of chatbot would you like to build? Let us know in the comments below!
PythonProgramming #ChatbotDevelopment #LearnPython #AIForBeginners #PythonTips #NLTK #ChatbotTutorial #CodingMadeSimple #PythonChatbot #TechBlog #ProgrammingTutorial #PythonHacks #AIProjects #CodeNewbie #MachineLearningBasics
Happy coding! 🐍